copied
Readme
Files and versions
1.6 KiB
Face Embeddings using Deepface
Author: Krishna katyal
Description
The pipeline is used to extract the feature vector of detected faces in images. It uses the for face embeddings Deepface
.
Code Example
Load an image from path './test_face'.
Write the pipeline in simplified style:
import towhee
towhee.glob('./test_face.jpg') \
.image_decode.cv2() \
.face_embedding.deepface(model_name = 'DeepFace').tolist()
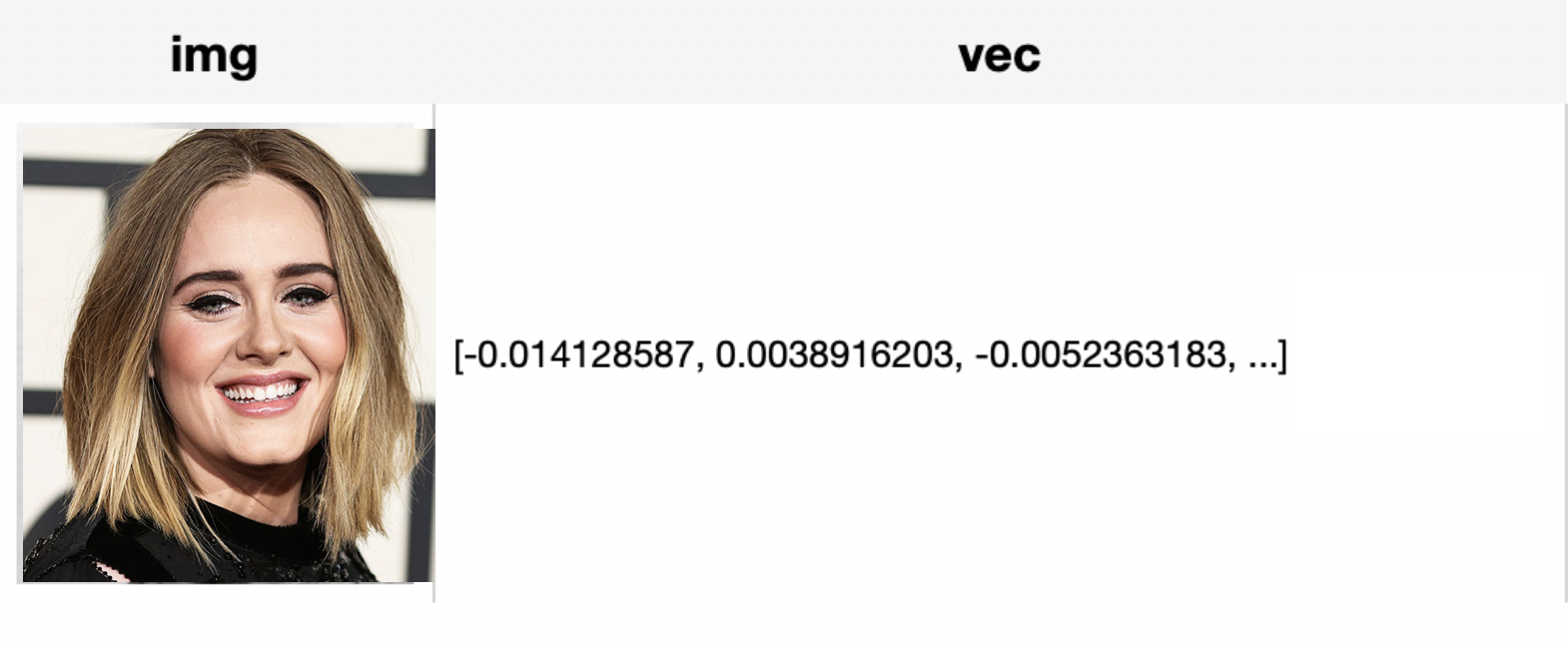
Write a pipeline with explicit inputs/outputs name specifications:
import towhee
towhee.glob['path']('./test_face.jpgg') \
.image_decode.cv2['path', 'img']() \
.face_embedding.deepface['img', 'vec']() \
.select['img','vec']() \
.show()
Factory Constructor
Create the operator via the following factory method
face_embedding.deepface(model_name = 'which model to use')
Model options:
- VGG-Face
- FaceNet
- OpenFace
- DeepFace
- ArcFace
- Dlib
- DeepID
Interface
A face embedding operator takes a face image as input. It extracts the embedding in ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The input image.
Returns: numpy.ndarray
The extracted image embedding.
Reference
https://github.com/serengil/deepface
You can refer to Getting Started with Towhee for more details. If you have any questions, you can submit an issue to the towhee repository.
1.6 KiB
Face Embeddings using Deepface
Author: Krishna katyal
Description
The pipeline is used to extract the feature vector of detected faces in images. It uses the for face embeddings Deepface
.
Code Example
Load an image from path './test_face'.
Write the pipeline in simplified style:
import towhee
towhee.glob('./test_face.jpg') \
.image_decode.cv2() \
.face_embedding.deepface(model_name = 'DeepFace').tolist()
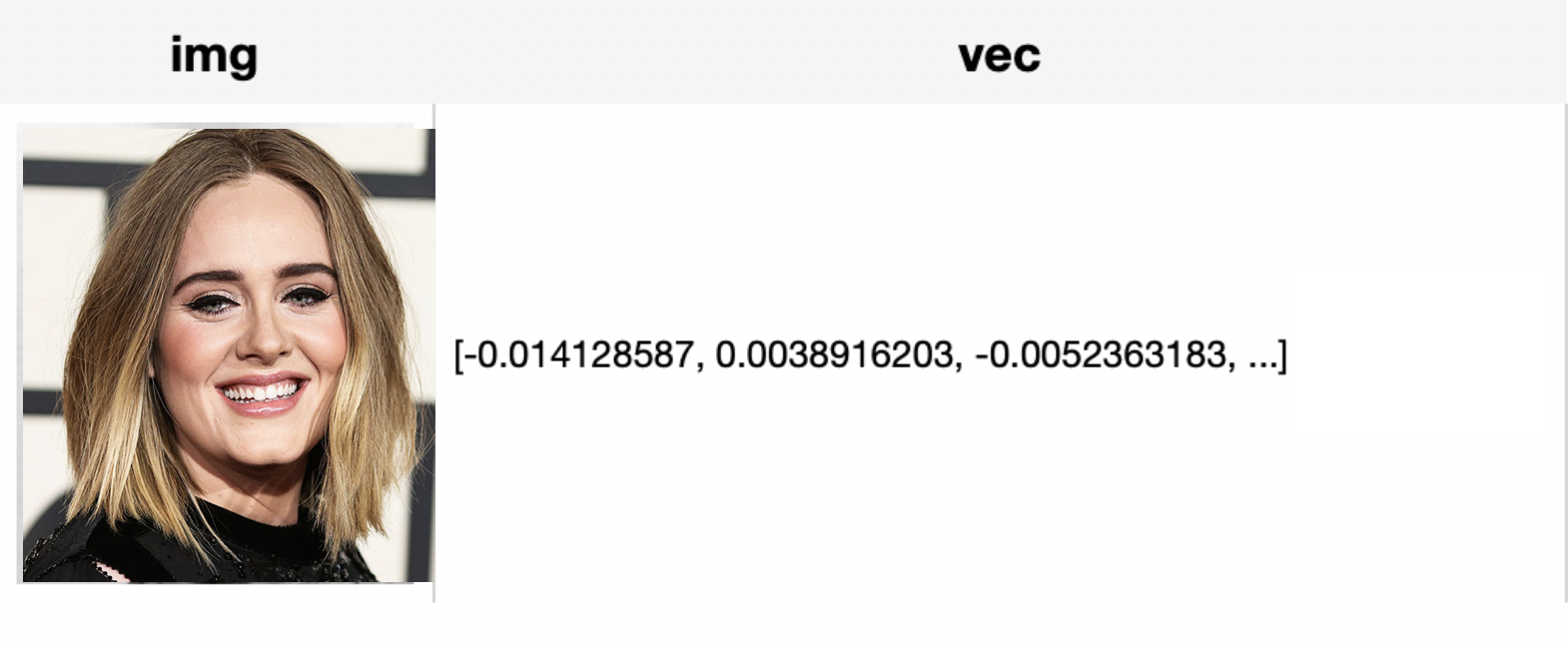
Write a pipeline with explicit inputs/outputs name specifications:
import towhee
towhee.glob['path']('./test_face.jpgg') \
.image_decode.cv2['path', 'img']() \
.face_embedding.deepface['img', 'vec']() \
.select['img','vec']() \
.show()
Factory Constructor
Create the operator via the following factory method
face_embedding.deepface(model_name = 'which model to use')
Model options:
- VGG-Face
- FaceNet
- OpenFace
- DeepFace
- ArcFace
- Dlib
- DeepID
Interface
A face embedding operator takes a face image as input. It extracts the embedding in ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The input image.
Returns: numpy.ndarray
The extracted image embedding.
Reference
https://github.com/serengil/deepface
You can refer to Getting Started with Towhee for more details. If you have any questions, you can submit an issue to the towhee repository.