copied
Readme
Files and versions
3.6 KiB
Inception-ResNet v1 Face Embedding Operator
author: David Wang
Description
This operator extracts embedding vector from facial image using Inception-ResNet. The implementation is an adaptation from timesler/facenet-pytorch.
Code Example
Extract face image embedding from './img.png'.
Write a pipeline with explicit inputs/outputs name specifications:
from towhee import pipe, ops, DataCollection
p = (
pipe.input('path')
.map('path', 'img', ops.image_decode())
.map('img', 'vec', ops.face_embedding.inceptionresnetv1())
.output('img', 'vec')
)
DataCollection(p('img.png')).show()
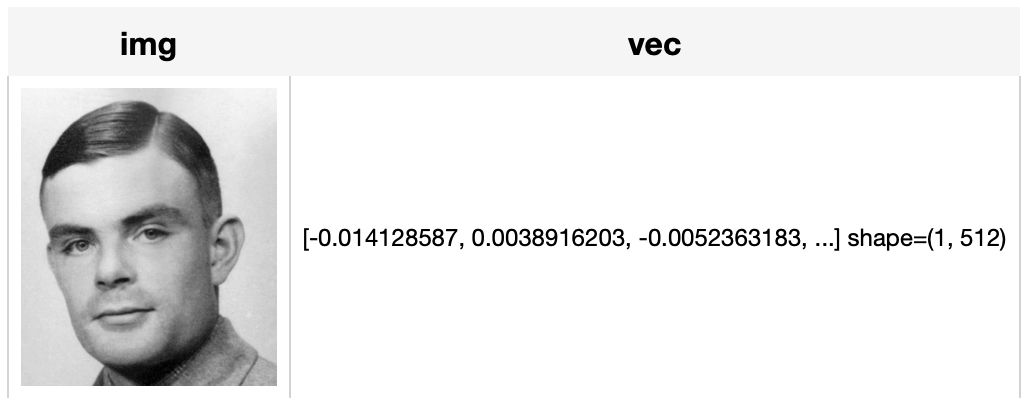
Factory Constructor
Create the operator via the following factory method:
face_embedding.inceptionresnetv1(image_size = 160)
Parameters:
image_size: int
Scaled input image size to extract embedding. The higher resolution would generate the more discriminative feature but cost more time to calculate.
supported types: int
, default is 160.
Interface
A face embedding operator takes a face image as input. It extracts the embedding in ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The input image.
Returns: numpy.ndarray
The extracted image embedding.
More Resources
- Exploring Multimodal Embeddings with FiftyOne and Milvus - Zilliz blog: This post explored how multimodal embeddings work with Voxel51 and Milvus.
- How to Get the Right Vector Embeddings - Zilliz blog: A comprehensive introduction to vector embeddings and how to generate them with popular open-source models.
- The guide to clip-vit-base-patch32 | OpenAI: clip-vit-base-patch32: a CLIP multimodal model variant by OpenAI for image and text embedding.
- Understanding ImageNet: A Key Resource for Computer Vision and AI Research: The large-scale image database with over 14 million annotated images. Learn how this dataset supports advancements in computer vision.
- Sparse and Dense Embeddings: A Guide for Effective Information Retrieval with Milvus | Zilliz Webinar: Zilliz webinar covering what sparse and dense embeddings are and when you'd want to use one over the other.
- Understanding Neural Network Embeddings - Zilliz blog: This article is dedicated to going a bit more in-depth into embeddings/embedding vectors, along with how they are used in modern ML algorithms and pipelines.
- Image Embeddings for Enhanced Image Search - Zilliz blog: Image Embeddings are the core of modern computer vision algorithms. Understand their implementation and use cases and explore different image embedding models.
- Enhancing Information Retrieval with Sparse Embeddings | Zilliz Learn - Zilliz blog: Explore the inner workings, advantages, and practical applications of learned sparse embeddings with the Milvus vector database
3.6 KiB
Inception-ResNet v1 Face Embedding Operator
author: David Wang
Description
This operator extracts embedding vector from facial image using Inception-ResNet. The implementation is an adaptation from timesler/facenet-pytorch.
Code Example
Extract face image embedding from './img.png'.
Write a pipeline with explicit inputs/outputs name specifications:
from towhee import pipe, ops, DataCollection
p = (
pipe.input('path')
.map('path', 'img', ops.image_decode())
.map('img', 'vec', ops.face_embedding.inceptionresnetv1())
.output('img', 'vec')
)
DataCollection(p('img.png')).show()
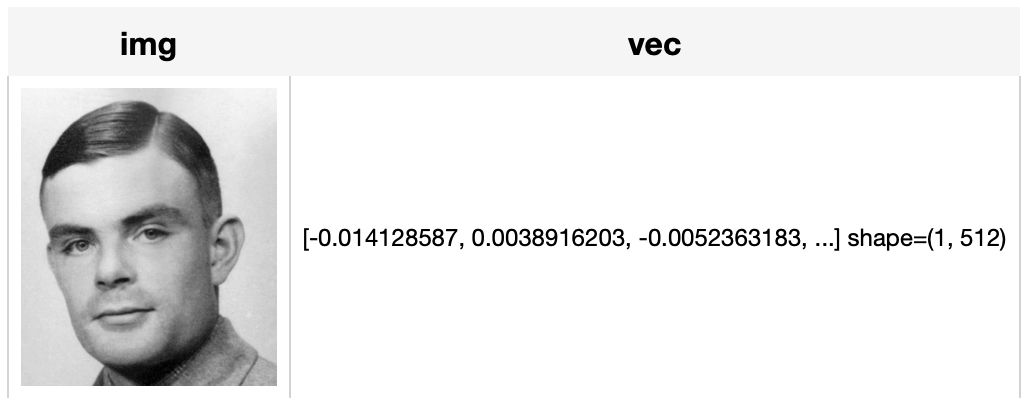
Factory Constructor
Create the operator via the following factory method:
face_embedding.inceptionresnetv1(image_size = 160)
Parameters:
image_size: int
Scaled input image size to extract embedding. The higher resolution would generate the more discriminative feature but cost more time to calculate.
supported types: int
, default is 160.
Interface
A face embedding operator takes a face image as input. It extracts the embedding in ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The input image.
Returns: numpy.ndarray
The extracted image embedding.
More Resources
- Exploring Multimodal Embeddings with FiftyOne and Milvus - Zilliz blog: This post explored how multimodal embeddings work with Voxel51 and Milvus.
- How to Get the Right Vector Embeddings - Zilliz blog: A comprehensive introduction to vector embeddings and how to generate them with popular open-source models.
- The guide to clip-vit-base-patch32 | OpenAI: clip-vit-base-patch32: a CLIP multimodal model variant by OpenAI for image and text embedding.
- Understanding ImageNet: A Key Resource for Computer Vision and AI Research: The large-scale image database with over 14 million annotated images. Learn how this dataset supports advancements in computer vision.
- Sparse and Dense Embeddings: A Guide for Effective Information Retrieval with Milvus | Zilliz Webinar: Zilliz webinar covering what sparse and dense embeddings are and when you'd want to use one over the other.
- Understanding Neural Network Embeddings - Zilliz blog: This article is dedicated to going a bit more in-depth into embeddings/embedding vectors, along with how they are used in modern ML algorithms and pipelines.
- Image Embeddings for Enhanced Image Search - Zilliz blog: Image Embeddings are the core of modern computer vision algorithms. Understand their implementation and use cases and explore different image embedding models.
- Enhancing Information Retrieval with Sparse Embeddings | Zilliz Learn - Zilliz blog: Explore the inner workings, advantages, and practical applications of learned sparse embeddings with the Milvus vector database