copied
Readme
Files and versions
3.3 KiB
MobileFaceNet Face Landmark Detector
author: David Wang
Description
MobileFaceNets is a class of extremely efficient CNN models to extract 68 landmarks from a facial image. It use less than 1 million parameters and is specifically tailored for high-accuracy real-time face verification on mobile and embedded devices. This repository is an adaptation from cuijian/pytorch_face_landmark.
Code Example
Extract facial landmarks from './img1.jpg'.
Write a pipeline with explicit inputs/outputs name specifications:
from towhee import pipe, ops, DataCollection
p = (
pipe.input('path')
.map('path', 'img', ops.image_decode())
.map('img', 'landmark', ops.face_landmark_detection.mobilefacenet())
.output('img', 'landmark')
)
DataCollection(p('./img1.jpg')).show()
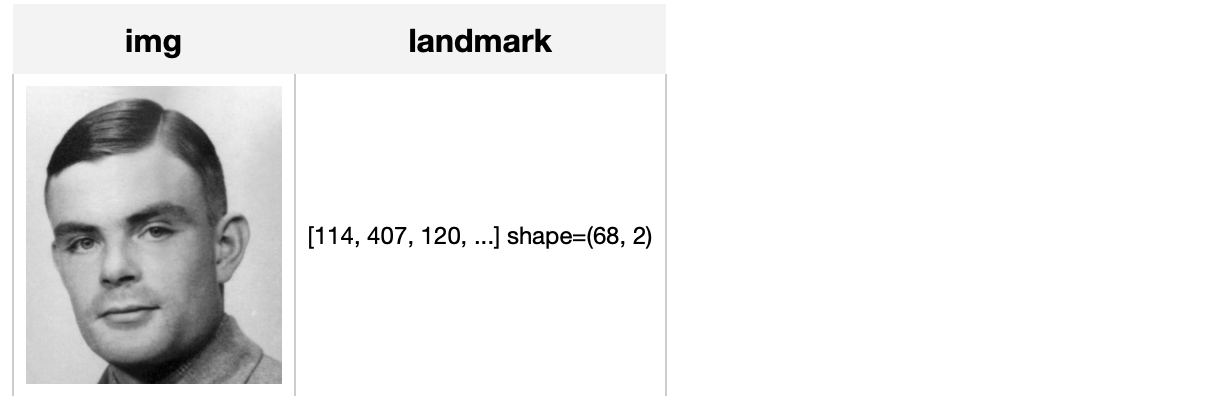
Factory Constructor
Create the operator via the following factory method:
face_landmark_detection.mobilefacenet(pretrained = True)
Parameters:
pretrained
whether load the pre-trained weights.
supported types: bool
, default is True, using pre-trained weights.
Interface
An image embedding operator takes an image as input. it extracts the embedding as ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The input image.
Returns: numpy.ndarray
The extracted facial landmarks.
More Resources
- Approximate Nearest Neighbors Oh Yeah (Annoy) - Zilliz blog: Discover the capabilities of Annoy, an innovative algorithm revolutionizing approximate nearest neighbor searches for enhanced efficiency and precision.
- How to Get the Right Vector Embeddings - Zilliz blog: A comprehensive introduction to vector embeddings and how to generate them with popular open-source models.
- Hugging Face Inference Endpoints & Zilliz Cloud: nan
- Transforming Text: The Rise of Sentence Transformers in NLP - Zilliz blog: Everything you need to know about the Transformers model, exploring its architecture, implementation, and limitations
- Understanding ImageNet: A Key Resource for Computer Vision and AI Research: The large-scale image database with over 14 million annotated images. Learn how this dataset supports advancements in computer vision.
- Build a Multimodal Search System with Milvus - Zilliz blog: Implementing a Multimodal Similarity Search System Using Milvus, Radient, ImageBind, and Meta-Chameleon-7b
- Zilliz-Hugging Face partnership - Explore transformer data model repo: Use Hugging Faceâs community-driven repository of data models to convert unstructured data into embeddings to store in Zilliz Cloud, and access a code tutorial.
3.3 KiB
MobileFaceNet Face Landmark Detector
author: David Wang
Description
MobileFaceNets is a class of extremely efficient CNN models to extract 68 landmarks from a facial image. It use less than 1 million parameters and is specifically tailored for high-accuracy real-time face verification on mobile and embedded devices. This repository is an adaptation from cuijian/pytorch_face_landmark.
Code Example
Extract facial landmarks from './img1.jpg'.
Write a pipeline with explicit inputs/outputs name specifications:
from towhee import pipe, ops, DataCollection
p = (
pipe.input('path')
.map('path', 'img', ops.image_decode())
.map('img', 'landmark', ops.face_landmark_detection.mobilefacenet())
.output('img', 'landmark')
)
DataCollection(p('./img1.jpg')).show()
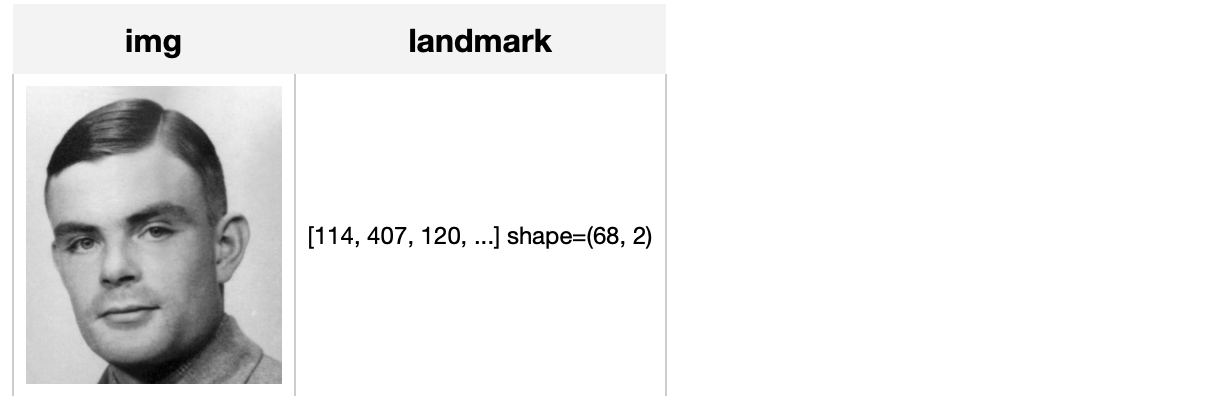
Factory Constructor
Create the operator via the following factory method:
face_landmark_detection.mobilefacenet(pretrained = True)
Parameters:
pretrained
whether load the pre-trained weights.
supported types: bool
, default is True, using pre-trained weights.
Interface
An image embedding operator takes an image as input. it extracts the embedding as ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The input image.
Returns: numpy.ndarray
The extracted facial landmarks.
More Resources
- Approximate Nearest Neighbors Oh Yeah (Annoy) - Zilliz blog: Discover the capabilities of Annoy, an innovative algorithm revolutionizing approximate nearest neighbor searches for enhanced efficiency and precision.
- How to Get the Right Vector Embeddings - Zilliz blog: A comprehensive introduction to vector embeddings and how to generate them with popular open-source models.
- Hugging Face Inference Endpoints & Zilliz Cloud: nan
- Transforming Text: The Rise of Sentence Transformers in NLP - Zilliz blog: Everything you need to know about the Transformers model, exploring its architecture, implementation, and limitations
- Understanding ImageNet: A Key Resource for Computer Vision and AI Research: The large-scale image database with over 14 million annotated images. Learn how this dataset supports advancements in computer vision.
- Build a Multimodal Search System with Milvus - Zilliz blog: Implementing a Multimodal Similarity Search System Using Milvus, Radient, ImageBind, and Meta-Chameleon-7b
- Zilliz-Hugging Face partnership - Explore transformer data model repo: Use Hugging Faceâs community-driven repository of data models to convert unstructured data into embeddings to store in Zilliz Cloud, and access a code tutorial.