copied
Readme
Files and versions
1.8 KiB
Image Captioning with ClipCap
author: David Wang
Description
This operator generates the caption with ClipCap which describes the content of the given image. ClipCap uses CLIP encoding as a prefix to the caption, by employing a simple mapping network, and then fine-tunes a language model to generate the image captions. This is an adaptation from rmokady/CLIP_prefix_caption.
Code Example
Load an image from path './hulk.jpg' to generate the caption.
Write the pipeline in simplified style:
import towhee
towhee.glob('./hulk.jpg') \
.image_decode() \
.image_captioning.clipcap(model_name='clipcap_coco') \
.show()

Write a same pipeline with explicit inputs/outputs name specifications:
import towhee
towhee.glob['path']('./hulk.jpg') \
.image_decode['path', 'img']() \
.image_captioning.clipcap['img', 'text'](model_name='clipcap_coco') \
.select['img', 'text']() \
.show()
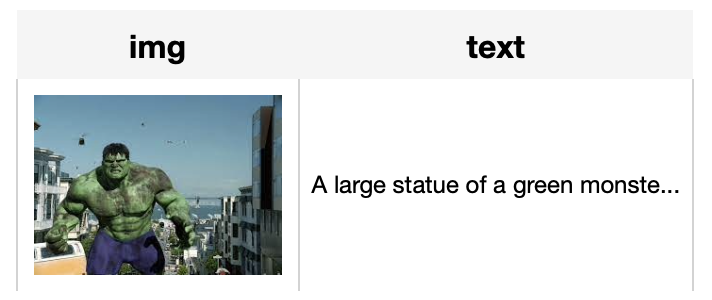
Factory Constructor
Create the operator via the following factory method
clipcap(model_name)
Parameters:
model_name: str
The model name of ClipCap. Supported model names:
- clipcap_coco
- clipcap_conceptual
Interface
An image captioning operator takes a towhee image as input and generate the correspoing caption.
Parameters:
data: towhee.types.Image (a sub-class of numpy.ndarray)
The image to generate caption.
Returns: str
The caption generated by model.
1.8 KiB
Image Captioning with ClipCap
author: David Wang
Description
This operator generates the caption with ClipCap which describes the content of the given image. ClipCap uses CLIP encoding as a prefix to the caption, by employing a simple mapping network, and then fine-tunes a language model to generate the image captions. This is an adaptation from rmokady/CLIP_prefix_caption.
Code Example
Load an image from path './hulk.jpg' to generate the caption.
Write the pipeline in simplified style:
import towhee
towhee.glob('./hulk.jpg') \
.image_decode() \
.image_captioning.clipcap(model_name='clipcap_coco') \
.show()

Write a same pipeline with explicit inputs/outputs name specifications:
import towhee
towhee.glob['path']('./hulk.jpg') \
.image_decode['path', 'img']() \
.image_captioning.clipcap['img', 'text'](model_name='clipcap_coco') \
.select['img', 'text']() \
.show()
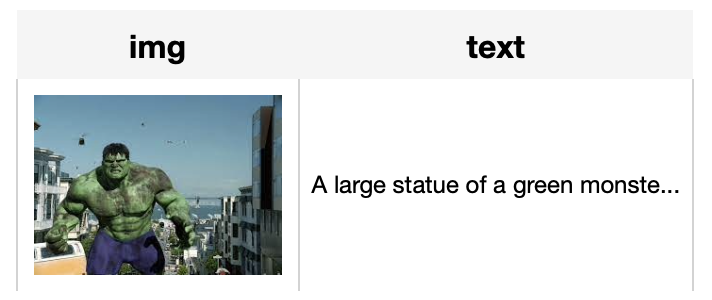
Factory Constructor
Create the operator via the following factory method
clipcap(model_name)
Parameters:
model_name: str
The model name of ClipCap. Supported model names:
- clipcap_coco
- clipcap_conceptual
Interface
An image captioning operator takes a towhee image as input and generate the correspoing caption.
Parameters:
data: towhee.types.Image (a sub-class of numpy.ndarray)
The image to generate caption.
Returns: str
The caption generated by model.