# Select video
*author: Chen Zhang*
## Description
This operator select input list by simply aggregating, sorting and then filtering it.
## Code Example
For two input lists: video urls and scores, aggregate url by reduce_function, then sort aggregated scores, then select top k results.
```python
import towhee
towhee.dc['video_urls', 'scores']([[['a', 'a', 'c', 'a', 'b', 'b', 'c', 'c'], [2, 8, 9.3, 5, 2, 1, 0, -1]]])\
.video_copy_detection.select_video[('video_urls','scores'), 'res'](top_k=2, reduce_function='mean', reverse=True)\
.show()
```
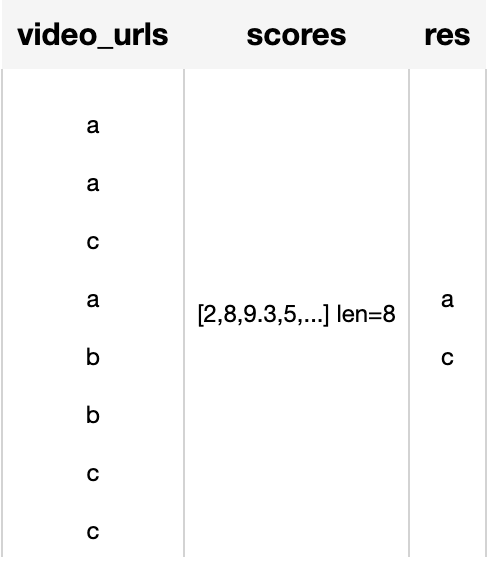
```python
import towhee
towhee.dc['video_urls', 'scores']([[['a', 'a', 'c', 'a', 'b', 'b', 'c', 'c'], [2, 8, 9.3, 5, 2, 1, 0, -1]]])\
.video_copy_detection.select_video[('video_urls','scores'), 'res'](top_k=2, reduce_function='sum', reverse=True)\
.show()
```
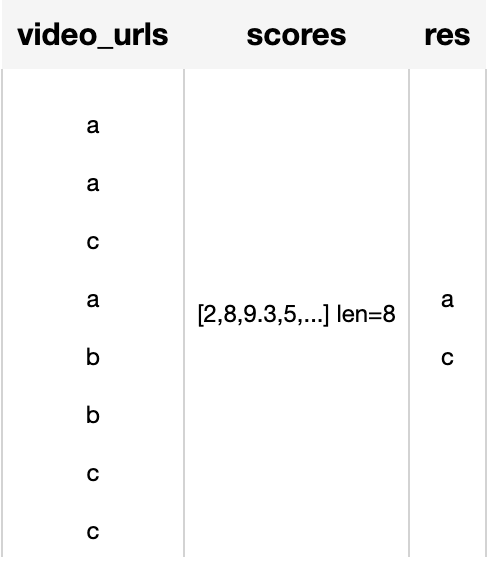
```python
import towhee
towhee.dc['video_urls', 'scores']([[['a', 'a', 'c', 'a', 'b', 'b', 'c', 'c'], [2, 8, 9.3, 5, 2, 1, 0, -1]]])\
.video_copy_detection.select_video[('video_urls','scores'), 'res'](top_k=2, reduce_function='max', reverse=True)\
.show()
```
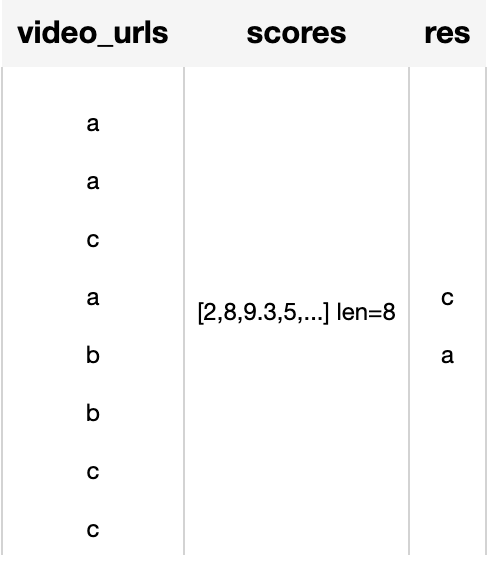
```python
import towhee
towhee.dc['video_urls', 'scores']([[['a', 'a', 'c', 'a', 'b', 'b', 'c', 'c'], [2, 8, 9.3, 5, 2, 1, 0, -1]]])\
.video_copy_detection.select_video[('video_urls','scores'), 'res'](top_k=2, reduce_function='min', reverse=True)\
.show()
```
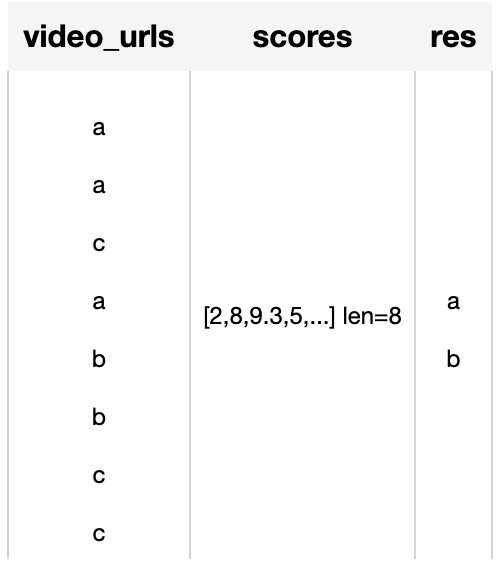
## Factory Constructor
Create the operator via the following factory method
***select_video(top_k: int, reduce_function: str, reverse: bool)***
**Parameters:**
***top_k:*** *int*
Select top k result.
***reduce_function:*** *str*
Aggregate function name, support name:
- sum
- mean
- max
- min
***reverse:*** *bool*
Whether sorted result is reversed.
## Interface
**Parameters:**
***video_urls:*** *List[str]*
Video urls.
***scores:*** *List[float]*
Every video scores.
**Returns:** *List[str]*
Selected video url results.