copied
Readme
Files and versions
Updated 3 years ago
image-embedding
Image Embedding with Timm
author: Jael Gu, Filip
Description
An image embedding operator generates a vector given an image. This operator extracts features for image with pre-trained models provided by Timm. Timm is a deep-learning library developed by Ross Wightman, who maintains SOTA deep-learning models and tools in computer vision.
Code Example
Load an image from path './towhee.jpeg' and use the pre-trained ResNet50 model ('resnet50') to generate an image embedding.
Write the pipeline in simplified style:
import towhee
towhee.glob('./towhee.jpeg') \
.image_decode() \
.image_embedding.timm(model_name='resnet50') \
.show()

Write a same pipeline with explicit inputs/outputs name specifications:
import towhee
towhee.glob['path']('./towhee.jpeg') \
.image_decode['path', 'img']() \
.image_embedding.timm['img', 'vec'](model_name='resnet50') \
.select['img', 'vec']() \
.show()
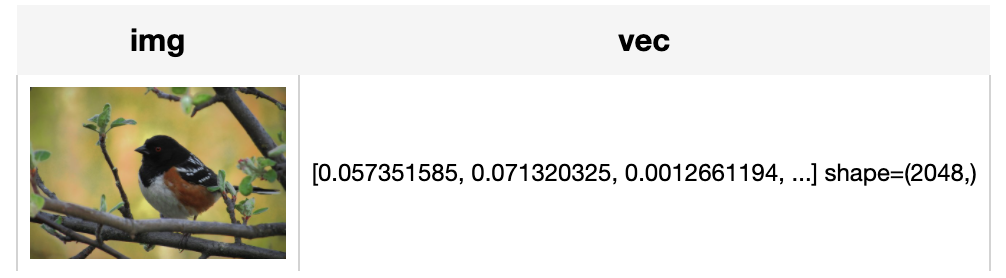
Factory Constructor
Create the operator via the following factory method:
image_embedding.timm(model_name='resnet34', num_classes=1000, skip_preprocess=False)
Parameters:
model_name: str
The model name in string. The default value is "resnet34". Refer to Timm Docs to get a full list of supported models.
num_classes: int
The number of classes. The default value is 1000. It is related to model and dataset.
skip_preprocess: bool
The flag to control whether to skip image pre-process. The default value is False. If set to True, it will skip image preprocessing steps (transforms). In this case, input image data must be prepared in advance in order to properly fit the model.
Interface
An image embedding operator takes a towhee image as input. It uses the pre-trained model specified by model name to generate an image embedding in ndarray.
Parameters:
img: towhee.types.Image (a sub-class of numpy.ndarray)
The decoded image data in numpy.ndarray.
Returns: numpy.ndarray
The image embedding extracted by model.
| 47 Commits | ||
---|---|---|---|
|
1.1 KiB
|
3 years ago | |
|
2.3 KiB
|
3 years ago | |
|
680 B
|
3 years ago | |
|
31 B
|
3 years ago | |
|
4.0 KiB
|
3 years ago | |
|
80 KiB
|
3 years ago | |
|
2.5 KiB
|
3 years ago | |
|
2.5 KiB
|
3 years ago | |
|
4.8 KiB
|
3 years ago | |
|
49 KiB
|
3 years ago |